Wednesday, December 29, 2010
Tuesday, December 28, 2010
String Format for Double
Tutorial Link: Local Project: http://www.csharp-examples.net/string-format-double/
Digits after decimal point
This example formats double to string with fixed number of decimal places. For two decimal places use pattern „0.00“. If a float number has less decimal places, the rest digits on the right will be zeroes. If it has more decimal places, the number will be rounded.
Next example formats double to string with floating number of decimal places. E.g. for maximal two decimal places use pattern „0.##“.
Digits before decimal point
If you want a float number to have any minimal number of digits before decimal point use N-times zero before decimal point. E.g. pattern „00.0“ formats a float number to string with at least two digits before decimal point and one digit after that.
Align numbers with spaces
To align float number to the right use comma „,“ option before the colon. Type comma followed by a number of spaces, e.g. „0,10:0.0“ (this can be used only in String.Format method, not in double.ToString method). To align numbers to the left use negative number of spaces.
Digits after decimal point
This example formats double to string with fixed number of decimal places. For two decimal places use pattern „0.00“. If a float number has less decimal places, the rest digits on the right will be zeroes. If it has more decimal places, the number will be rounded.
Next example formats double to string with floating number of decimal places. E.g. for maximal two decimal places use pattern „0.##“.
Digits before decimal point
If you want a float number to have any minimal number of digits before decimal point use N-times zero before decimal point. E.g. pattern „00.0“ formats a float number to string with at least two digits before decimal point and one digit after that.
Align numbers with spaces
To align float number to the right use comma „,“ option before the colon. Type comma followed by a number of spaces, e.g. „0,10:0.0“ (this can be used only in String.Format method, not in double.ToString method). To align numbers to the left use negative number of spaces.
Sunday, December 19, 2010
Create an automated screenshot
Original Link: http://www.grasshopper3d.com/forum/topics/having-grasshopper-create-an
Rectangle bounds = Screen.GetBounds(System.Drawing.Point.Empty);
using(Bitmap bitmap = new Bitmap(bounds.Width, bounds.Height))
{
using(Graphics g = Graphics.FromImage((Image) bitmap))
{
g.CopyFromScreen(System.Drawing.Point.Empty, System.Drawing.Point.Empty, bounds.Size);
}
bitmap.Save(@"C:\SomePath\SomeFilename.jpg", System.Drawing.Imaging.ImageFormat.Jpeg);
}
Concave Hall
paid application: http://www.concavehull.com/home.php?main_menu=1
Local Project: http://ubicomp.algoritmi.uminho.pt/local/
algorithm: http://www.concavehull.com/home.php?main_menu=1
Online application: http://local.dsi.uminho.pt:8080/webMathematica/ConcaveHull/index.html
Saturday, December 18, 2010
DataTree in C#
How to create a DataTree in C# using Grasshopper:
DataTree
for (int i = 0; i <= y; i++){
// Adding data to a DataTree requires a path.
// In this case the path will have a single branch index.
// There will be only one Point associated with each path
Point3d pt = new Point3d(i, 0, 0);
EH_Path Path = new EH_Path(i);
Tree.Add(pt, Path);
}
Friday, December 10, 2010
how to port Excel data to GH
original source link
Dim xlApp As Object
''Grab a running instance of Excel
xlApp = System.Runtime.InteropServices.Marshal.GetActiveObject("Excel.Application")
''send volume and area to Excel
xlApp.range("B12").Value = volume
xlApp.range("B13").Value = area
Thursday, December 9, 2010
PQ Mesh
Reference from EAT-A-BUG:
http://eat-a-bug.blogspot.com/2010/09/planar-quad-mesh-design.html
http://eat-a-bug.blogspot.com/2010/09/planar-quad-mesh-design-pt-2.html
This is a exercise to create a Tool to generate a quad-dorminate mesh with only planar faces only--PQ Mesh, which was first presented in this paper.


Sunday, October 31, 2010
Iterate through all objects in Grasshopper Document
Namespace: Grasshopper.Kernel
object class : GH_Document
Attributes : public IList
// this return all the objects(components) in the Grasshopper file.

Friday, October 15, 2010
Display.DisplayPipeline RhinoCommon
from David Rutten: link
Dim cd As New Rhino.Display.CustomDisplay(True)
cd.AddPoint(New Point3d(0,0,0))
cd.AddPoint(New Point3d(1,0,0), Color.DarkRed, PointStyle.Simple, 5)
cd.AddLine(New Line(New Point(0,0,0), New Point(1,0,0)), Color.DarkGreen, 4)
cd.AddVector(New Point3d(5,5,0), New Vector3d(0,0,10), Color.OrangeRed, True)
'from this point onwards the viewports will show the above geometry.
cd.Dispose()
'Once Dispose has been called the preview will be cleared. You can no longer use this instance of CustomDisplay from now on.
Thursday, October 14, 2010
TKU PARAMETRIC URBAN FURNITURE
TKU PARAMETRIC URBAN FURNITURE - workshop in 2010 Jan
with Yuan & Goerge @ TKU, Taiwan
http://tkupufworkshop.blogspot.com/
with Yuan & Goerge @ TKU, Taiwan
http://tkupufworkshop.blogspot.com/
Wednesday, October 13, 2010
UbiMash - PROTOTYPING RESPONSIVE MODELS WITH PHYSICAL COMPUTING AND PARAMETRIC DESIGN
Tuesday, October 12, 2010
Polyline Network Surface on Rhino
An example of using Rhinoscript for creating a Netwrok Surface in Rhino:
Original Link: Polyline Network Surface
Option Explicit
‘Script written by Howard Jiho Kim
‘Script version Monday, March 10, 2008 12:46:21 PM
Call Main()
Sub Main()
Dim strObject, strObject2, arrObjects
Dim netCrv(100)
Dim i
Dim arrNew
i=0
arrObjects = Rhino.GetObjects(“Pick some curves”, 4)
If IsArray(arrObjects) Then
For Each strObject In arrObjects
If Rhino.IsPolyline(strObject) Then
netCrv(i) = SubDividePolyline(strObject)
Print netCrv(i)
Rhino.SelectObject netCrv(i)
i=i+1
End If
Next
End If
Rhino.Command(“NetworkSrf”)
End Sub
Function SubDividePolyline(strObject)
Dim arrV
arrV = Rhino.PolylineVertices(strObject)
Dim arrSubD() : ReDim arrSubD(2 * UBound(arrV))
Dim i
For i=0 To UBound(arrV)-1
arrSubD(i*2) = arrV(i)
arrSubD(i*2+1) = Array( (arrV(i)(0) + arrV(i+1)(0))/ 2.0, _
(arrV(i)(1) + arrV(i+1)(1))/ 2.0, _
(arrV(i)(2) + arrV(i+1)(2))/ 2.0)
Next
arrSubD(UBound(arrSubD)) = arrV(UBound(arrV))
SubDividePolyline = Rhino.AddCurve(arrSubD)
End Function
Thursday, October 7, 2010
Realtime Physics for Space Planning
Original Link from Grasshopper3D: Realtime Physics for Space Planning
vedio:http://vimeo.com/15563685
Tuesday, October 5, 2010
Freeform Origami | Software
A very interesting application:
This software is a design software that allows users to interact with origami forms while altering the crease pattern of the model. The software can keep:
Developability (foldable from a piece of paper)
Flat-foldability (foldable into a flat shape)
Planarity of facets (paper do not twist in 3D form)
Point coordinate coincidence
Paper size
Available on September 2010
http://www.youtube.com/watch?v=T35So_88mio&feature=player_embedded
This software is a design software that allows users to interact with origami forms while altering the crease pattern of the model. The software can keep:
Developability (foldable from a piece of paper)
Flat-foldability (foldable into a flat shape)
Planarity of facets (paper do not twist in 3D form)
Point coordinate coincidence
Paper size
Available on September 2010
http://www.youtube.com/watch?v=T35So_88mio&feature=player_embedded
Wednesday, September 29, 2010
Backe an object with an assigned name
Bake an object with the assigned name from Grasshopper : Backe an object with an assigned name
file fro m Grasshopper3D: AddNameToObj.ghx, 31 KB
if (BakeNow)
{
Rhino.DocObjects.ObjectAttributes att = new Rhino.DocObjects.ObjectAttributes();
att.Name = NewName;
//If it's a brep, a polysurface or a surface
if (Obj is Brep)
{
doc.Objects.AddBrep((Brep) Obj, att);
A = "Brep baked";
}
//If it's a mesh
else if (Obj is Mesh)
{
doc.Objects.AddMesh((Mesh) Obj, att);
A = "Mesh baked";
}
//If it's a line (not a curve)
else if (Obj is Line)
{
doc.Objects.AddLine((Line) Obj, att);
A = "Line baked";
}
//If it's an arc (not a curve)
else if (Obj is Arc)
{
doc.Objects.AddArc((Arc) Obj, att);
A = "Arc baked";
}
//If it's a circle (not a curve)
else if (Obj is Circle)
{
doc.Objects.AddCircle((Circle) Obj, att);
A = "Circle baked";
}
//If it's a curve)
else if (Obj is Curve)
{
doc.Objects.AddCurve((Curve) Obj, att);
A = "Curve baked";
}
//If it's a Point3D)
else if (Obj is Point3d)
{
doc.Objects.AddPoint((Point3d) Obj, att);
A = "Point baked";
}
else
{
A = "Don't Know how to handle this geometry.";
}
}
Tuesday, September 28, 2010
From "Geometry and New and Future Spatial Patterns" by Pottman, H. (2009). Architectural Design. M. Garcia, Wiley. 79: 60–65.
The architects/designers do not always have the right tools at their disposal to realize such structures.
The realization of complex architecture freeform shapes and generation of panel patterns continues to be especially challenging, limited by material and manufacturing constraints.
The architects/designers do not always have the right tools at their disposal to realize such structures.
The realization of complex architecture freeform shapes and generation of panel patterns continues to be especially challenging, limited by material and manufacturing constraints.
Friday, September 24, 2010
Create a Parametric Helix in Revit
Two Steps:
1. Create a line family, which has parameters for the vertical offset, the length of the line segment and rotation angle.

2. insert number of this line objects into another mass template for the creation of the helix.

Wednesday, September 8, 2010
About Optimization from David
Original link: forum/topics/learning-vbnet-and-rhinocommon
optimization comes in multiple flavours. Macro-Optimization is difficult but generally yields good results, especially if you're implementing proven algorithms (binary search vs. linear search for example). Micro-Optimization rarely makes a big difference, especially when you're using 'smart' compilers like modern C++ or .NET ones. In fact, it's not that rare that an attempt at micro-optimization is actually detrimental, as the compiler suddenly no longer 'understands' what it is you're doing and can no longer help out.
Two things I've learned over the years about optimization:
1. You will not be able to guess/deduce which parts of your code are bottlenecks. Don't start blindly optimizing code.
Always always always profile your code first
. You can either build in custom profilers (the .NET framework supplies some useful classes for this) or use an external profiler (I use RedGate Ants).2. Debugging code is twice as hard as writing code. Therefore, writing complicated optimizations that stretch your skills to the limit is pointless and frustrating. You will not be able to debug your optimized code and end up worse off than when you started.
Saturday, September 4, 2010
Friday, September 3, 2010
Voronoi 3D from Grasshopper3D
Tutorial Link:http://www.grasshopper3d.com/forum/topics/3d-voronoi-1
Tutorial from un didi:
http://dimitrie.wordpress.com/2009/05/01/3d-voronoi-in-grasshopper/
updated version: http://improved.ro/Grasshopper/Voronoi3dAndQconvex.zip
Qhull : http://www.qhull.org/
Automatic Differentiation
A C++ library that is unlicensed and freely available that performs AD is posted below. A discussion from Grasshopper3D.
Links:
http://www.grasshopper3d.com/forum/topics/gh-feature-request-automatic
Attachment: Fast Automatic Differentiation in C++.zip
http://www.codeproject.com/KB/cpp/fadcpp.aspx
Thursday, September 2, 2010
Backing Objects with colors
Link: http://www.grasshopper3d.com/forum/topics/error-in-old-vb-code
If blnBake Then
'Bake Object
Dim mObj As New MRhinoBrepObject
mObj = doc.AddBrepObject(obj)
'Find obj attributes to change color
Dim att As New MRhinoObjectAttributes(mObj.Attributes())
att.SetColorSource(1) 'set color source to From Object
Dim rColor As New OnColor (color) 'make OnColor
att.m_color = rColor 'Set Object Color
'Modify the attributes
Dim objref As New MRhinoObjRef(mObj.Attributes.m_uuid)
doc.ModifyObjectAttributes(objref, att)
End If
If blnBake Then
'Bake Object
Dim mObj As New MRhinoBrepObject
mObj = doc.AddBrepObject(obj)
'Find obj attributes to change color
Dim att As New MRhinoObjectAttributes(mObj.Attributes())
att.SetColorSource(1) 'set color source to From Object
Dim rColor As New OnColor (color) 'make OnColor
att.m_color = rColor 'Set Object Color
'Modify the attributes
Dim objref As New MRhinoObjRef(mObj.Attributes.m_uuid)
doc.ModifyObjectAttributes(objref, att)
End If
Path Mapper
from David:
The PathMapper is a very complicated piece of work, thousands of lines of code go into evaluating and parsing the mapping masks. You can however use PathMapper logic from your own code. What you need to do is create a
GH_LexerCombo
which contains the source and target mappings. Then you can callGH_Lexer.PerformLexicalReplace()
to map a data tree.link:http://www.grasshopper3d.com/forum/topics/hi-everyone-help-me-please-on
Friday, August 27, 2010
Isopotential Maps by C#
An attempt to build the Isopotential Maps from the tutorial below:
Tutorial Link: http://www.grasshopper3d.com/profiles/blogs/generating-isopotential-maps
Definition:

Perspective:
Tutorial Link: http://www.grasshopper3d.com/profiles/blogs/generating-isopotential-maps
Definition:

Perspective:

Wednesday, August 18, 2010
buildz_Orthagonal on Placement: making a brick wall
Another tutorial from Buildz shows how to create a brick wall by Revit Curtain wall and customized brick family.
Tutorial Link: http://buildz.blogspot.com/2010/07/orthogonal-on-placement-making-brick.html
image from buildz:

Sun Tracking Panels
An example of using the "Orientation" parameter for adaptive points, particularly "orthogonal on placement". Using the same features, you can design a panel that creates optimized relationships to solar orientation regardless of where it is placed on a particular surface.
Tutorial Link: http://buildz.blogspot.com/2010/08/look-ma-no-api-making-sun-tracker.html
Vedio from http://buildz.blogspot.com/:
Tutorial Link: http://buildz.blogspot.com/2010/08/look-ma-no-api-making-sun-tracker.html
Vedio from http://buildz.blogspot.com/:
Friday, August 13, 2010
CopyTrimmed
RhinoCommon:
to be updated:
CreateTrimmedSurface or CopyTrimCurves may be what you are looking for
M_Rhino_Geometry_Brep_CreateTrimmedSurface.htm
M_Rhino_Geometry_Brep_CopyTrimCurves.htm
Keep tracking the new release of RhinoCommon for the Brep attributes for additional parametric control.
Rhino 4.0 .NET SDK:
----------------------------------------------------------------------
OnBrep copyTrimmedPattern(OnBrep source, OnSurface target)
{
OnBrep brep = new OnBrep(source);
if((brep.m_F.Count() == 1) && target != null)
{
OnBrepFace face = brep.m_F[0];
OnSurface surface = target.DuplicateSurface();
int index = brep.AddSurface(surface);
face.ChangeSurface(index);
if (brep.RebuildEdges(ref face, 0.001, true, true))
{
brep.CullUnusedSurfaces();
}
}
return brep;
}
----------------------------------------------------------------------
2010 0810 CopyTrim:
to be updated:
CreateTrimmedSurface or CopyTrimCurves may be what you are looking for
M_Rhino_Geometry_Brep_CreateTrimmedSurface.htm
M_Rhino_Geometry_Brep_CopyTrimCurves.htm
Keep tracking the new release of RhinoCommon for the Brep attributes for additional parametric control.
Rhino 4.0 .NET SDK:
----------------------------------------------------------------------
OnBrep copyTrimmedPattern(OnBrep source, OnSurface target)
{
OnBrep brep = new OnBrep(source);
if((brep.m_F.Count() == 1) && target != null)
{
OnBrepFace face = brep.m_F[0];
OnSurface surface = target.DuplicateSurface();
int index = brep.AddSurface(surface);
face.ChangeSurface(index);
if (brep.RebuildEdges(ref face, 0.001, true, true))
{
brep.CullUnusedSurfaces();
}
}
return brep;
}
----------------------------------------------------------------------
2010 0810 CopyTrim:
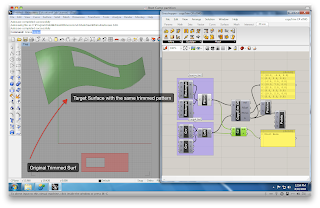
Monday, July 26, 2010
Run RhinoScript in C# component in Grasshopper
To run a Rhinoscript in C# component in Grasshopper:
for example:
RMA.Rhino.RhUtil.RhinoApp().RunScript("-_Render");
for example:
RMA.Rhino.RhUtil.RhinoApp().RunScript("-_Render");
Sunday, July 18, 2010
open URL
GH3D VB example: http://www.grasshopper3d.com/forum/topics/open-url-from-vb
.Net Reference: http://msdn.microsoft.com/en-us/library/system.net.webclient(VS.80).aspx
image from GH3D:

another example: reading data from a web site
private void RunScript(string url, ref object A)
{
System.Net.WebClient client = new System.Net.WebClient();
A = client.DownloadString(url);
}
image from GH3D:
.Net Reference: http://msdn.microsoft.com/en-us/library/system.net.webclient(VS.80).aspx
image from GH3D:
another example: reading data from a web site
private void RunScript(string url, ref object A)
{
System.Net.WebClient client = new System.Net.WebClient();
A = client.DownloadString(url);
}
image from GH3D:

Friday, July 16, 2010
Thursday, July 15, 2010
Irregular Grid
Think about the following examples from GH3D:



GH3D Links:
1. unevenly-divided-surface
2. irregular-diagrid



GH3D Links:
1. unevenly-divided-surface
2. irregular-diagrid
Switch UV coordinates
GH3D Link:
http://www.grasshopper3d.com/forum/topics/switch-uv-coordinates
1. Scripting:
If srf Is Nothing Then
Return
End If
srf.Transpose()
A = srf
2. using component:
http://www.grasshopper3d.com/forum/topics/switch-uv-coordinates
1. Scripting:
If srf Is Nothing Then
Return
End If
srf.Transpose()
A = srf
2. using component:
GH2Revit, Digital Project
original GH3D link: export-to-digital-project
http://www.grasshopper3d.com/forum/topics/export-to-digital-project
Grasshopper3d to Autodesk Robot - CableNet:
http://www.geometrygym.blogspot.com/
from GH to Revit via CSV file:
http://theprovingground.wikidot.com/revit-csv
http://www.grasshopper3d.com/forum/topics/export-to-digital-project
Grasshopper3d to Autodesk Robot - CableNet:
http://www.geometrygym.blogspot.com/
from GH to Revit via CSV file:
http://theprovingground.wikidot.com/revit-csv
Image data from Viewport
Link : http://www.grasshopper3d.com/forum/topics/how-to-get-the-image-data-such
Private Sub RunScript(ByRef A As Object)
RMA.Rhino.RhUtil.RhinoApp().RunScript("-_ViewCaptureToClipboard _Enter")
Dim img As Image = Windows.Forms.Clipboard.GetImage()
Dim bmp As New Bitmap(20, 20, Imaging.PixelFormat.Format24bppRgb)
Dim grp As Graphics = Graphics.FromImage(bmp)
grp.InterpolationMode = Drawing2D.InterpolationMode.HighQualityBicubic
grp.DrawImage(img, 0, 0, 20, 20)
Dim R As int32 = 0
Dim G As int32 = 0
Dim B As Int32 = 0
For i As Int32 = 0 To bmp.Width - 1
For j As Int32 = 0 To bmp.Height - 1
Dim col As Color = bmp.GetPixel(i, j)
R += col.R
G += col.G
B += col.B
Next
Next
R = Convert.ToInt32(R / 400)
G = Convert.ToInt32(G / 400)
B = Convert.ToInt32(B / 400)
A = Color.FromArgb(R, G, B)
End Sub
Screen Capture a Viewport
http://wiki.mcneel.com/developer/sdksamples/screencaptureview
Private Sub RunScript(ByRef A As Object)
RMA.Rhino.RhUtil.RhinoApp().RunScript("-_ViewCaptureToClipboard _Enter")
Dim img As Image = Windows.Forms.Clipboard.GetImage()
Dim bmp As New Bitmap(20, 20, Imaging.PixelFormat.Format24bppRgb)
Dim grp As Graphics = Graphics.FromImage(bmp)
grp.InterpolationMode = Drawing2D.InterpolationMode.HighQualityBicubic
grp.DrawImage(img, 0, 0, 20, 20)
Dim R As int32 = 0
Dim G As int32 = 0
Dim B As Int32 = 0
For i As Int32 = 0 To bmp.Width - 1
For j As Int32 = 0 To bmp.Height - 1
Dim col As Color = bmp.GetPixel(i, j)
R += col.R
G += col.G
B += col.B
Next
Next
R = Convert.ToInt32(R / 400)
G = Convert.ToInt32(G / 400)
B = Convert.ToInt32(B / 400)
A = Color.FromArgb(R, G, B)
End Sub
Screen Capture a Viewport
http://wiki.mcneel.com/developer/sdksamples/screencaptureview
Disable Customized Component Preview
In the constructor of the component, set the Hidden property to True.
C#:
public Component_Name()
: base("BlahBlahBlah", "Blah", "Life is visceral.", "Cat", "Pan")
{
this.Hidden = true;
}
Link: http://www.grasshopper3d.com/forum/topics/component-disabled-preview
C#:
public Component_Name()
: base("BlahBlahBlah", "Blah", "Life is visceral.", "Cat", "Pan")
{
this.Hidden = true;
}
Link: http://www.grasshopper3d.com/forum/topics/component-disabled-preview
Data Tree
have a look in these two examples - they show how to read or make a tree. Like Thomas is saying, you will need the component from here to open the file correctly.
Attachments: gh-trees.ghx, 61 KB
Attachments: gh-trees.ghx, 61 KB
Contour component with paths
GH3D links: http://www.grasshopper3d.com/forum/topics/suggestion-contour-component
Private Sub RunScript(ByVal S As OnGeometry, ByVal G As Double, ByVal D As OnLine, ByVal L As Boolean, ByRef C As Object, ByRef P As Object)
If S IsNot Nothing AndAlso G > 0 Then
Dim continp As New MRhinoContourInput
Dim GeoArr(0) As OnGeometry
GeoArr(0) = S
continp.m_geom = GeoArr
continp.m_interval = G
If D IsNot Nothing Then
continp.m_basept = D.from
continp.m_endpt = D.To
Else
continp.m_basept = New On3dPoint(0, 0, 0)
continp.m_endpt = New On3dPoint(0, 0, 1)
End If
continp.m_limit_range = L
continp.m_JoinCurves = 1
Dim Crvs() As OnCurve = Nothing
Dim pline() As OnPolyline = Nothing
Dim pts As ArrayOn3dPoint = Nothing
Dim check As New Boolean
check = RhUtil.MakeRhinoContours(continp, pline, Crvs, pts)
If check Then
C = Crvs
P = pline
Else print("Contour Failed. SDK function returned an Error.")
End If
Else print("Invalid/Null Input(s)")
End If
End Sub
Private Sub RunScript(ByVal S As OnGeometry, ByVal G As Double, ByVal D As OnLine, ByVal L As Boolean, ByRef C As Object, ByRef P As Object)
If S IsNot Nothing AndAlso G > 0 Then
Dim continp As New MRhinoContourInput
Dim GeoArr(0) As OnGeometry
GeoArr(0) = S
continp.m_geom = GeoArr
continp.m_interval = G
If D IsNot Nothing Then
continp.m_basept = D.from
continp.m_endpt = D.To
Else
continp.m_basept = New On3dPoint(0, 0, 0)
continp.m_endpt = New On3dPoint(0, 0, 1)
End If
continp.m_limit_range = L
continp.m_JoinCurves = 1
Dim Crvs() As OnCurve = Nothing
Dim pline() As OnPolyline = Nothing
Dim pts As ArrayOn3dPoint = Nothing
Dim check As New Boolean
check = RhUtil.MakeRhinoContours(continp, pline, Crvs, pts)
If check Then
C = Crvs
P = pline
Else print("Contour Failed. SDK function returned an Error.")
End If
Else print("Invalid/Null Input(s)")
End If
End Sub
objects by Layers
GH3D links:
more can be found : Rhino .NET Framework SDK
Bake object to specific layers:
Private Sub RunScript(ByVal obj As Brep, ByVal lay As String, ByVal col As Color, ByRef A As Object)
Dim lay_index As Int32 = doc.Layers.Find(lay, True)
If (lay_index < 0) Then
lay_index = doc.Layers.Add(lay, col)
End If
Dim att As New DocObjects.ObjectAttributes()
att.LayerIndex = lay_index
A = doc.Objects.AddBrep(obj, att)
End Sub
Subscribe to:
Posts (Atom)